Students can refer to the ICSE Class 10 Computer Applications Notes given below prepared as per the latest syllabus and examination guidelines issued for ICSE Class 10 Computer Applications. As this is a very scoring subject, students of ICSE are suggested to refer to the important revision notes below and use them to understand all critical topics in the chapters, this will help you to get better marks in exams.
ICSE Class 10 Computer Applications Notes
Please refer to the following chapter-wise notes for Computer Applications in ICSE Standard 10th. These revision notes have been prepared based on the latest examination pattern so that you are able to revise the entire course prior to the examinations. These notes have been prepared by our expert faculty from major ICSE schools in India.
Chapter – 1 : Revision of Class-IX Syllabus
Quick Review
* Java is an Object-Oriented Language.
* As a language that has the Object-Oriented feature, Java supports the following fundamental concepts.
(i) Polymorphism : Ability of an object to take on many forms.
(ii) Inheritance : A mechanism in which one object acquires all the properties and behaviours of parent class.
(iii) Encapsulation : A process of wrapping code and data together into a single unit i.e., class.
(iv) Abstraction : Process of hiding the implementation details from the user.
* Object : Objects have states and behaviours. Example: A dog has states – colour, name, breed as well as behaviours like – wagging the tail, barking, eating etc. An object is an instance of a class.
* Class : A class can be defined as a template/blueprint that describes the behaviour/state that the object of its type support.
* Instance : An object is an instance of a class.
* Method : A method is a collection of statements that are grouped together to perform the specified task.
Know the Terms
* Local variables : Variables defined inside methods, constructors or blocks are called local variables. The variable will be declared and initialized within the method and the variable will be destroyed when the method has completed.
* Instance variables : Instance variables are variables within a class but outside any method. These variables are initialized when the class is instantiated. Instance variables can be accessed from inside any method, constructor or blocks of that particular class.
* Class variables : Class variables are variables declared within a class, outside any method, with the static keyword.
Glossary
AWT : Abstract Window Toolkit
JVM : Java Virtual Machine
JIT : Just In Time
JDT : Java Development Toolkit
API : Application Programming Interface
Chapter – 2 : Call as the Basis of all Computation
Quick Review
* There are two data types available in Java :
(i) Primitive Data Types
(ii) Reference/Object Data Types
* Primitive Data Types
There are eight primitive data-types supported by Java. Primitive data-types are predefined by the language and named by a keyword. Let us now look into the eight primitive data types in detail.
(i) byte
(a) Byte data type is an 8-bit signed two’s complement integer.
(b) Minimum value is -128 (-2^7).
(c) Maximum value is 127 (inclusive)(2^7 -1).
(d) Default value is 0.
(e) Byte data type is used to save space in large arrays, mainly in place of integers, since a byte is four times smaller than an integer.
(f) Example, byte a = 100, byte b = -50.
(ii) short
(a) Short data type is a 16-bit signed two’s complement integer.
(b) Minimum value is -32,768 (-2^15).
(c) Maximum value is 32,767 (inclusive) (2^15 -1).
(d) Short data type can also be used to save memory as byte data type. A short is 2 times smaller than an integer.
(e) Default value is 0.
(f) Example, short s = 10000, short r = -20000.
(iii) int
(a) int data type is a 32-bit signed two’s complement integer.
(b) Minimum value is – 2,147,483,648 (-2^31).
(c) Maximum value is 2,147,483,647(inclusive) (2^31 -1).
(d) Integer is generally used as the default data type for integral values unless there is a concern about memory.
(e) The default value is 0.
(f) Example, int a = 100000, int b = -200000.
(iv) long
(a) Long data type is a 64-bit signed two’s complement integer.
(b) Minimum value is -9,223,372,036,854,775,808(-2^63).
(c) Maximum value is 9,223,372,036,854,775,807 (inclusive)(2^63 -1).
(d) This type is used when a wider range than int is needed.
(e) Default value is 0L.
(f) Example, long a = 100000L, long b = -200000L.
(v) float
(a) Float data type is a single-precision 32-bit IEEE 754 floating point.
(b) Float is mainly used to save memory in large arrays of floating point numbers.
(c) Default value is 0.0f.
(d) Float data type is never used for precise values such as currency.
(e) Example, float f1 = 234.5f.
(vi) double
(a) double data type is a double-precision 64-bit IEEE 754 floating point.
(b) This data type is generally used as the default data type for decimal values, generally the default choice.
(c) Double data type should never be used for precise values such as currency.
(d) Default value is 0.0d.
(e) Example, double d1 = 123.4.
(vii) boolean
(a) boolean data type represents one bit of information.
(b) There are only two possible values: true and false.
(c) This data type is used for simple flags that track true/false conditions.
(d) Default value is false.
(e) Example, boolean one = true.
(viii) char
(a) char data type is a single 16-bit Unicode character.
(b) Minimum value is ‘\u0000’ (or 0).
(c) Maximum value is ‘\uffff ’ (or 65,535 inclusive).
(d) Char data type is used to store any character.
(e) Example, char letterA = ‘A’.
* Reference Data-types
(i) Reference variables are created using defined constructors of the classes. They are used to access objects. These variables are declared to be of a specific type that cannot be changed. For example, Employee, Puppy, etc.
(ii) Class objects and various type of array variables come under reference data-type.
(iii) Default value of any reference variable is null.
(iv) A reference variable can be used to refer any object of the declared type or any compatible type.
Know the Terms
Access modifiers :
Each object has members (members can be data variable and methods) which can be declared to have specific access. Java has 4 access level and 3 access modifiers. Access levels are listed below in the least to most restrictive order.
* Public : Members (variables, methods, and constructors) declared public (least restrictive) within a public class are visible to any class in the Java program, whether these classes are in the same package or in another package.
* Protected : The protected fields or methods, cannot be used for classes and Interfaces. Fields, methods and constructors declared protected in a super-class can be accessed only by subclasses in other packages. Classes in the same package can also access protected fields, methods and constructors as well, even if they are not a subclass of the protected member ’s class.
* Private : The private (most restrictive) modifiers can be used for members but cannot be used for classes and Interfaces. Fields, methods or constructors declared private are strictly controlled, which means they cannot be accessed by anywhere outside the enclosing class.
Glossary
Byte Code : Java portable code
Applet : Small Java Program
Bean : Java Software
Void : Null Value
Template : Generic Class
Chapter – 3 : Constructors
Quick Review
* Constructor in Java is a special type of method that is used to initialize the object.
* Java constructor is invoked at the time of object creation. It constructs the values i.e. provides data for the object that is why it is known as constructor.
* Rules for creating Java Constructor :
There are basically two rules defined for the constructor.
(i) Constructor name must be same as its class name.
(ii) Constructor must have no explicit return type.
* Types of Java Constructors :
There are following types of constructors.
(i) Default constructor (no-argument constructor)
(ii) Parameterised constructor
(iii) Constructor with Arguments
(iv) Constructor overloading
Know the Terms
* Default Constructor – It is the no argument constructor that is automatically created by compiler in the absence of explicit constructor.
* Parameterised Constructor – These constructors are required to pass parameters on creation of objects.
* Constructor with Arguments – It is possible to define constructors with argument.
* Constructor Overloading – Constructor overloading is the way of having more than one constructor, which does a various tasks.
The number of parameters can be same also but it can have different data types.
Glossary
New : Java Unary Operator
Token : Internal Value Assigned
Separators : Comma and Terminators
Signatures : Function calling parameters
Chapter – 4 : Functions
Quick Review
* Function : A program module used simultaneously at different instances in the program is called method or function.
* Defining a Function :
<Access specifier><Return type><method name>(parameter list)
{• //Body of the function•}
(i) Access specifier – public or private.
(ii) Method declared without access specifier is by default treated as public
(iii) Type- Specifies the data of the value returned from the method
(iv) Function name – Preferably related to the program
(v) Parameter list – Variables which receives the value passed from the arguments during method call
* Components of a method : There are two components –
(i) Header ( also known as method prototype)
(ii) Body List of parameters is called signature
* Header public int add() Body return(value). The statement which sends back the value from a method is called program return statement and is used at the end of the program which is a function terminator.
* Types of Functions :
(i) Pure function, also called accessor.
(ii) Impure function, also called mutator.
* Pure function or Accessor takes objects as an arguments but does not modify the state of the objects.
* Impure function or mutator takes objects on an argument and charges the state of the objects.
* Different ways of defining a Function :
(i) Receiving value and returning outcome to the caller.
(ii) Receiving values but not returning outcome to the caller and vice versa.
(iii) Neither receiving values nor returning outcome to the caller.
* Formal parameter :
(i) Parameter is a variable used with method signature that receives a value during function call.
(ii) When the parameter values are received from the caller then it is called Formal parameter.
* Actual Parameter : If the values are passed to the method during its call from the caller then it is actual parameter
* Pass by value :
(i) Process of passing a copy of actual arguments to the formal parameters.
(ii) Any change made in the formal will not reflect on actual argument.
* Formal parameters after function operation Actual arguments before function call X Y A B 7 8 5 6 Actual arguments after function call A B Copied formal parameters during function call 5 6 X Y 5 6.
* Pass by Reference :
(i) Process of passing the reference of actual arguments to the formal parameters and any change in the formal parameters will be reflected in the actual parameters also
* Function overloading :
(i) Function with the same name but used for different purposes is called as overloaded functions.
(ii) It is implemented through polymorphism.
(iii) Compiler finds the best match of the function arguments and the parameter list during program compilation called static binding.
* Recursive function :
(i) A function which calls by itself is called as recursive function.
Chapter – 5 : Class as a user Defined Type
Quick Review
* There are 8 primitive data types defind in Java, they are byte, short, long, float, double, char, boolean.
* Java allows us to create new data type using combination of the primitive data types, such data types are called composite or user-defined data types.
* Just as variables can be declared of any primitive data type, similarly we can declare variables of the composite data types.
* Class is an example of composite data type and we can declare objects as variables of the class.
* Following are some of the differences between primitive data types and user-defined data types :
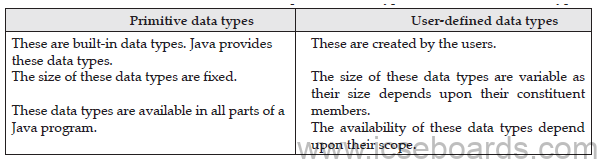
* Access modifiers :
public – methods of the same class and methods of other classes
private – methods of the same class only
protected – methods of the same class, methods of subclasses, and methods of classes in the same package
none – methods in the same package only
* Value-returning methods
Use a return statement to return the value i.e., return expression;
(i)Each of Java’s eight primitive data types has a class dedicated to it. These are known as wrapper classes.
(ii)The most common methods of the Integer wrapper class are summarized in below table. Similar methods for the other wrapper classes are found in the Java API documentation.
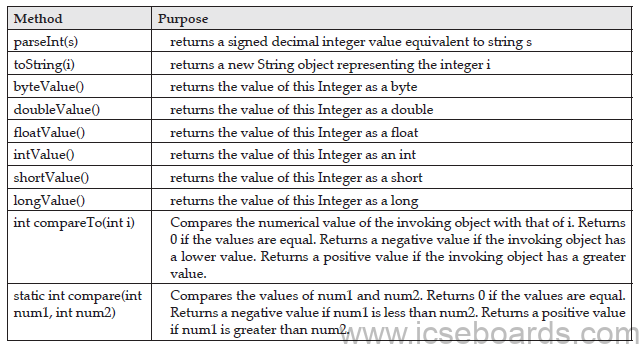
Know the Terms
* Client of a class : A program that instantiates objects and calls methods of the class.
* Fields : A variable that resides within the class, and whose scope is to the entire class.
* Instance variables : data for each object –
Class Data : static data that all objects shared.
* Class Members : fields and methods, within the class
* Access Modifiers : determines access rights for the class and its members – defines where the class and its members can be used.
Chapter – 6 : Iterations
Quick Review
* Iteration statements are statements (or compound statements) to be executed zero or more times, subject to some loop-termination criteria. When these statements are compound statements, they are executed in order, except when either the break statement or the continue statement is encountered.
* Loops are basically means to do a task multiple times, without actually coding all statements over and over again.
For example, loops can be used for displaying a string many times, for counting numbers and of course for displaying menus.
* Loops in Java are mainly of three types :
- ‘while’ loop : A while statement executes its statements as long as a specified condition evaluates to true.
Syntax : While (condition)
statements. - The ‘do-while’ loop : It is very similar to the ‘while’ loop shown above. The only difference being, in a ‘while’ loop is that the condition is checked before the body of loop, but in a ‘do-while’ loop, the condition is checked after one execution of the loop.
Syntax : do statements
while (condition); - The ‘for ’ loop :
This is probably the most useful and the most used loop in Java The syntax is slightly more complicated than that of ‘while’ or the ‘do-while’ loop.
Syntax : for(<initial value>;<condition>;<increment/decrement>)
statements.
* The Break Statement :
Use the break statement to terminate a loop, switch, or in conjunction with a labelled statement.
(i) When you use break without a label, it terminates the innermost enclosing while, do-while, for, or switch immediately and transfers control to the following statement.
(ii) When you use break with a label, it terminates the specified labelled statement.
The syntax of the break statement looks like this :
break [Label]
* The Continue Statement :
The continue statement can be used to restart a while, do-while, for or label statement.
(i) When you use continue without a label, it terminates the current iteration of the innermost enclosing while, do-while, or for statement and continues execution of the loop with the next iteration. In contrast to the break statement, continue does not terminate the execution of the loop entirely. In a while loop, it jumps back to the condition. In a for loop, it jumps to the increment-expression.
(ii) When you use continue with a label, it applies to the looping statement identified with that label.
The syntax of the Continue statement looks like the following :
continue [Label];
Chapter – 7 : Using Library Classes
Quick Review
* The Java Development Kit comes with many libraries which contain predefined classes and methods to use.
These are standard Java classes or built-in Java classes, contained in packages.
These classes provide an effective support to the programmers in developing their program logic.
* Packages : The package contain a set of classes in order to ensure that class names are unique.
Some of these includes :
- a Math library (java.lang.Math).
- String library (java.lang.String).
- Graphics library (java.awt.* and javax.swing.*).
- Networking library (java.net).
Chapter -8 : Encapsulation
Quick Review
* Encapsulation is one of the four fundamental OOP concepts. The other three are inheritance, polymorphism, and abstraction.
* Encapsulation in Java is a mechanism of wrapping the data (variables) and code acting on the data (methods) together as a single unit. In encapsulation, the variables of a class will be hidden from other classes, and can be accessed only through the methods of their current class. Therefore, it is also known as data hiding.
* To achieve encapsulation in Java :
(i) Declare the variables of a class as private.
(ii) Provide public setter and getter methods to modify and view the variables values.
Benefits of Encapsulation :
* The fields of a class can be made read-only or write-only.
* A class can have total control over what is stored in its fields.
* The users of a class do not know how the class stores its data. A class can change the data type of a field and users of the class do not need to change any of their code.
Chapter -9: Arrays
Quick Review
* Java provides a data structure, the array, which stores a fixed-size sequential collection of elements of the same type. An array is used to store a collection of data, but it is often more useful to think of an array as a collection of variables of the same type.
* Elements of array will be referred as array-name [n], where n is the number of element in the array.
* Instead of declaring individual variables, such as number0, number1, …, and number99, you declare one array variable such as numbers and use numbers[0], numbers[1], and …, numbers[99] to represent individual variables.
* There are two types of array :
(i)Single Dimensional Array
(ii)Double Dimensional Array
* Array Creation : Syntax for array creation
array – name = new type [size];
where new is a keyword that allocates memory for an array.
* Declaring Array Variables :
• To use an array in a program, you must declare a variable to reference the array, and you must specify the type of array the variable can reference.
The syntax for declaring an array variable.
DataType arrayRef var [];
* Basic Operations on Arrays :
(i) Searching : It is the process to determine whether the given is present in the array or not. These are of two types –
(a) Linear Search
(b) Binary Search
(ii) Sorting : The process of arranging data in ascending or descending order. There are two ways to sort the data –
(a) Selection Sort
(b) Bubble Sort
Chapter -10 : Input / Output
Quick Review
* Input is any information needed by program to complete its execution while output is any information that the program must convey to the user.
* The input stream is used to read data from a source and the Output stream is used for writing data to a destination.
* There are various ways to read input from the keyboard, the java.util.scanner class is one of them.
* The scanner class allows the user to read values of various types.
* The syntax to create a scanner object is scanner input = new scanner (system.in);
* The Java scanner class breaks the input into tokens using a delimiter that is white space by default. It provides many methods to read and parse various primitive values.
* Java scanner class is widely used to parse text for string and primitive types using regular expression.
* Java scanner class extends object class and implements iterator and closeable interfaces.
* Methods for checking appearance of a specific token.
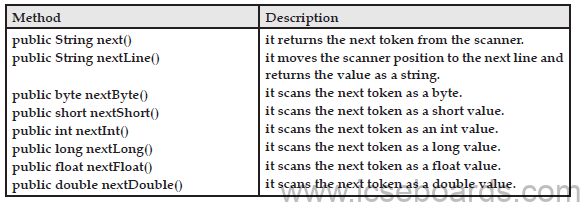
Student should refer to ICSE Class 10 Computer Applications Notes given above for all chapters in ICSE Class 10 Computer Applications. These revision notes have been prepared based on the latest syllabus and guidelines issued by ICSE. Class 10 students can refer to the above notes for Computer Applications which have been prepared keeping in consideration all the important topics which can be covered in the examinations and can be really scoring for students.
It is suggested by ICSE Class 10 Computer Applications teachers that the students should do a continuous revision of revision notes on a regular basis so that the pressure at the time of Computer Applications exam is reduced thus giving time to the student to concentrate on critical topics which can help them to get better marks in the exams. On our website we have provided here the best collection of revision notes which have been prepared by the best faculty in ICSE so that the students can download these Computer Applications notes and use them for revision to get better marks in their examinations.
The best way to read notes is by taking out print out and underlining the most important sentences which the student thinks is important or are difficult for the student to remember. On other pages of this website we have also provided important questions and Sample Papers for ICSE class 10 Computer Applications which have come in past examinations and which are teachers think are important and can be asked in future exams of ICSE Computer Applications class 10.
Students are suggested to download the question papers of Computer Applications ICSE Class 10 and practice them on a daily basis preferably in examination conditions at home which will help them to understand their weaknesses and strong points and it will help them to improve their ranking in the exams.
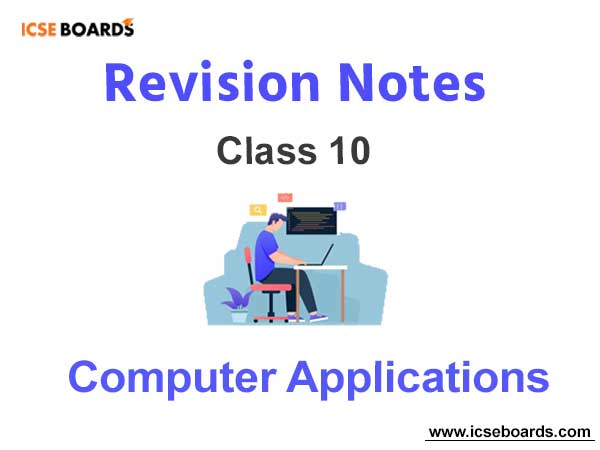
You can get free ICSE Class 10 Computer Applications Notes from https://www.icseboards.com/, All notes have been developed based on the latest syllabus and examination guidelines issued by ICSE
If you are looking for the latest revision notes for the Computer Applications subject in class 10 of ICSE then you have come to the right place as on our website we have provided the latest study material which has been really useful for ICSE students
We have provided all study material of ICSE on our website absolutely free. We do not charge anything from our students and readers.
Yes, all study material provided here on https://www.icseboards.com/ has been updated as per the latest syllabus issued by ICSE Board. We have made sure that all teachers have updated the content provided by them. If you refer to any section, such as sample papers or past year questions papers you will find everything updated.